7. Retrieval evidences¶
7.1. Notebook setup¶
%load_ext autoreload
%autoreload 2
import glob as glob
import matplotlib as mpl
import matplotlib.patheffects as PathEffects
import matplotlib.pyplot as plt
import matplotlib.transforms as transforms
import numpy as np
import pandas as pd
import seaborn as sns
import bz2
import corner
import json
import pathlib
import pickle
import utils
import warnings
from astropy import constants as const
from astropy import units as uni
from astropy.io import ascii, fits
from astropy.time import Time
from mpl_toolkits.axes_grid1 import ImageGrid
# Default figure dimensions
FIG_WIDE = (11, 5)
FIG_LARGE = (8, 11)
# Figure style
sns.set(style="ticks", palette="colorblind", color_codes=True, context="talk")
params = utils.plot_params()
plt.rcParams.update(params)
7.2. Dowload data¶
Unzip this into a folder named data
in the same level as this notebook
7.3. Load¶
base_dir_fixed_R0 = f"data/retrieval_evidence/spot_lower_fixed_R0"
(
df_Delta_lnZ_fixed,
df_Delta_lnZ_err_fixed,
species_min_fixed,
model_min_fixed,
data_dict_fixed,
) = utils.get_evidences(base_dir_fixed_R0)
base_dir_fit_R0 = f"data/retrieval_evidence/spot_upper_fit_R0"
(
df_Delta_lnZ_fit,
df_Delta_lnZ_err_fit,
species_min_fit,
model_min_fit,
data_dict_fit,
) = utils.get_evidences(base_dir_fit_R0)
print(f"Fixed R0: {base_dir_fixed_R0}")
print(f"Minimul log-evidence: ({species_min_fixed}, {model_min_fixed})")
display(df_Delta_lnZ_fixed)
print(f"\nFit R0: {base_dir_fit_R0}")
print(f"Minimul log-evidence: ({species_min_fit}, {model_min_fit})")
display(df_Delta_lnZ_fit)
Fixed R0: data/retrieval_evidence/spot_lower_fixed_R0
Minimul log-evidence: (K, clear)
Na | K | TiO | Na_K | Na_TiO | K_TiO | Na_K_TiO | |
---|---|---|---|---|---|---|---|
clear | 4.228008 | 0.000000 | 6.470431 | 3.961596 | 5.965399 | 5.906551 | 5.620903 |
clear+cloud | 3.631123 | 1.485933 | 5.398483 | 3.179458 | 5.170945 | 4.996205 | 4.870079 |
clear+haze | 3.684933 | 2.223177 | 5.362364 | 3.221512 | 5.124867 | 4.944949 | 4.720639 |
clear+cloud+haze | 2.848549 | 1.737777 | 4.479787 | 2.382097 | 4.248182 | 4.153051 | 3.856953 |
clear+spot | 4.241980 | 3.812417 | 4.241815 | 3.685709 | 3.997720 | 3.751752 | 3.506001 |
clear+spot+cloud | 3.174436 | 2.867850 | 3.291967 | 2.695057 | 3.033652 | 2.801135 | 2.538111 |
clear+spot+haze | 3.034682 | 2.817973 | 3.120746 | 2.614493 | 2.828451 | 2.655219 | 2.427965 |
clear+spot+cloud+haze | 2.159865 | 1.938158 | 2.208348 | 1.660904 | 1.910795 | 1.798888 | 1.529130 |
Fit R0: data/retrieval_evidence/spot_upper_fit_R0
Minimul log-evidence: (K, clear+spot+cloud)
Na | K | TiO | Na_K | Na_TiO | K_TiO | Na_K_TiO | |
---|---|---|---|---|---|---|---|
clear | 2.206913 | 0.380967 | 4.386723 | 2.023344 | 4.318345 | 4.282190 | 3.952981 |
clear+cloud | 1.678550 | 0.425468 | 3.723377 | 1.159673 | 3.509070 | 3.367628 | 3.187165 |
clear+haze | 1.984397 | 1.524298 | 3.549039 | 1.544969 | 3.454950 | 3.315019 | 3.104065 |
clear+cloud+haze | 1.355585 | 1.090464 | 2.813465 | 0.919345 | 2.765632 | 2.592734 | 2.307415 |
clear+spot | 1.873221 | 0.236316 | 4.110028 | 1.519822 | 3.922646 | 3.742836 | 3.549808 |
clear+spot+cloud | 1.157246 | 0.000000 | 3.388560 | 0.839379 | 3.148010 | 3.085014 | 2.661736 |
clear+spot+haze | 1.428711 | 1.113402 | 3.159281 | 1.112669 | 2.895913 | 2.834353 | 2.511222 |
clear+spot+cloud+haze | 0.855759 | 0.583121 | 2.396005 | 0.453123 | 2.027855 | 2.020561 | 1.619528 |
7.4. Plot¶
fig, axes = plt.subplots(1, 2, figsize=FIG_WIDE, sharey=True)
ax_left, ax_right = axes
# Left: Fixed R_0
p_fixed = utils.plot_evidence_summary(ax_left, df_Delta_lnZ_fixed)
p_fixed.annotate("Fixed $R_0$", xy=(0.05, 0.9), xycoords="axes fraction")
# Right: Fit R_0
p_fit = utils.plot_evidence_summary(ax_right, df_Delta_lnZ_fit)
p_fit.annotate("Fit $R_0$", xy=(0.05, 0.9), xycoords="axes fraction")
# From https://stackoverflow.com/a/55826518
bars = p_fixed.patches
# Possible hatch patterns: ['/', '\\', '|', '-', '+', 'x', 'o', 'O', '.', '*']
patterns = [
'/',
'\\',
'.',
'O',
] # set hatch patterns in the correct order
hatches = [] # list for hatches in the order of the bars
for h in patterns: # loop over patterns to create bar-ordered hatches
for i in range(int(len(bars) / len(patterns))):
hatches.append(h)
for bar, hatch in zip(bars, hatches): # loop over bars and hatches to set hatches in correct order
bar.set_hatch(hatch)
bars = p_fit.patches
# Possible hatch patterns: ['/', '\\', '|', '-', '+', 'x', 'o', 'O', '.', '*']
patterns = [
'/',
'\\',
'.',
'O',
] # set hatch patterns in the correct order
hatches = [] # list for hatches in the order of the bars
for h in patterns: # loop over patterns to create bar-ordered hatches
for i in range(int(len(bars) / len(patterns))):
hatches.append(h)
for bar, hatch in zip(bars, hatches): # loop over bars and hatches to set hatches in correct order
bar.set_hatch(hatch)
# Combined legend
handles, labels = ax_left.get_legend_handles_labels()
fig.legend(
handles,
labels,
loc="upper center",
ncol=4,
fontsize="12",
bbox_to_anchor=(0.5, 1.1),
)
# Save
fig.text(0.51, -0.02, "Species", ha="center")
fig.text(-0.01, 0.5, "Relative log-evidence", va="center", rotation="vertical")
fig.tight_layout()
fig.set_size_inches(FIG_WIDE)
utils.savefig(f"../paper/figures/retrieval_evidence/evidence_summary.pdf")
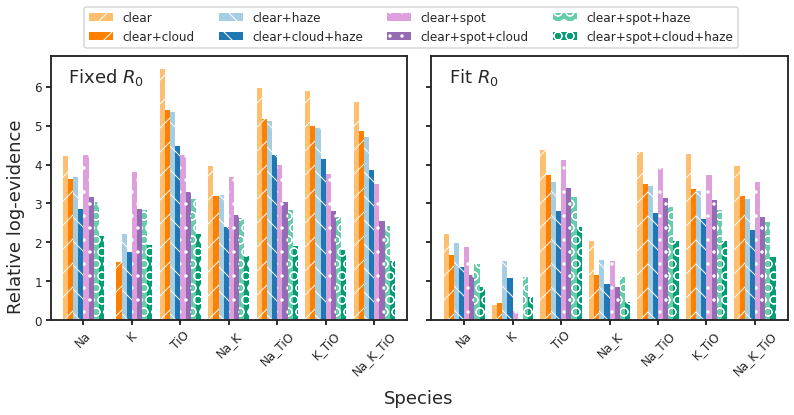